Scenario
You need the users to upload files to a specific directory for a temporary purpose. You dont want everyone to create an account. Just want to identify who uploaded for reference purpose only.
Requirements
PHP Server with a folder with Read / Write permission
We have used dropzone . js script for the drag and drop upload feature. [ dropzone dot dev ]
Features:
# Simple script
#drag and drop supported – User can upload single or multiple files
#any device or laptop or desktop or mobile supported
#enforce user to enter email id which will allow easy tracking of files as this will be prepended to the files uploaded.
Files :
- banner.jpg this can be a banner to have a nice looking interface. Dimension: 1329 X 180 pixels
- index.php – this file is the landing page
- upload.php – this is the file which actually moves the file from front end to the server.
- data : create this folder where script will upload the files
Codes
index.php
<!DOCTYPE html>
<html>
<head>
<title>Haneef File Uploader</title>
<!-- Bootstrap Css -->
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.4.1.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/dropzone/4.0.1/min/dropzone.min.css" rel="stylesheet">
<script src="https://cdnjs.cloudflare.com/ajax/libs/dropzone/4.2.0/min/dropzone.min.js"></script>
</head>
<body>
<div class="container">
<center><img src="banner.jpg" class="img-rounded img-fluid" alt="Cinque Terre"></center><hr>
<div class="row">
<div class="col-md-12">
<h2>Haneef File Uploader</h2>
<div class="row">
<div class="col"><div class="form-group">
<input type="email" class="form-control" id="email" aria-describedby="emailHelp" placeholder="Enter Email ID to proceed" onmouseout="clearfloor()">
</div></div>
<div class="col"><button type="button">Proceed</button></div>
</div>
<div id="uploadform">
<form action="upload.php" enctype="multipart/form-data" class="dropzone" id="image-upload">
<div>
<h3>Drop all Files here</h3>
</div>
<input type="hidden" name="fname" id="fname"/>
</form>
</div>
</div>
</div>
</div>
<script type="text/javascript">
$( document ).ready(function() {
$("#uploadform").hide( );
});
function validateEmail($email) {
var emailReg = /^([\w-\.]+@([\w-]+\.)+[\w-]{2,4})?$/;
return emailReg.test( $email );
}
function clearfloor(){
var k = $("#email").val();
if( !validateEmail(k)) {
alert('wrong email');
$("#uploadform").hide( );
}
else
{
$('input[name="fname"]').attr('value',k);
if(k == null || k == ''){
console.log('empty value');
}
else
{
$("#uploadform").show( );
}
}
}
Dropzone.autoDiscover = false;
var myDropzone = new Dropzone(".dropzone", {
autoProcessQueue: true,
maxFilesize: 3,
acceptedFiles: ".jpeg,.jpg,.png,.gif,.pdf,.doc.,docx"
});
$('#uploadFile').click(function(){
myDropzone.processQueue();
});
</script>
</body>
</html>
upload.php
<?php
$name = $_POST['fname'];
$uploadDir = 'data'; //Edit this line to define custom folder name
if (!empty($_FILES)) {
$tmpFile = $_FILES['file']['tmp_name'];
$filename = $uploadDir.'/'. $name.'-'.time().'-'. $_FILES['file']['name'];
move_uploaded_file($tmpFile,$filename);
}
?>
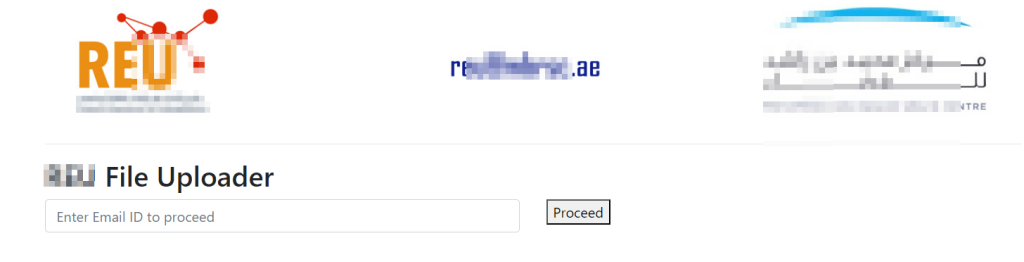
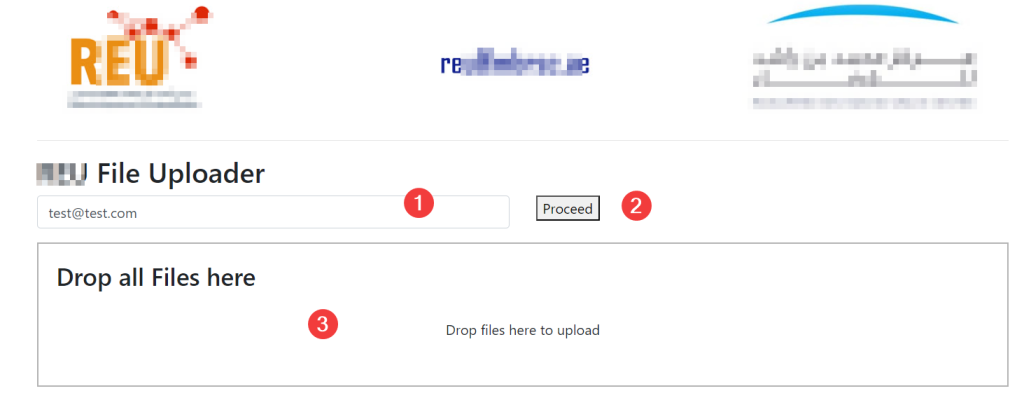
Notes :
File extensions can be supported in the index file by editing below line
acceptedFiles: “.jpeg,.jpg,.png,.gif,.pdf,.doc.,docx”
Upload folder path can be adjusted in upload.php folder
Make sure to give read / write permission to the folder.
Note that this is not a secure system however very simple system for quick file transfer between teams during any event / competitions etc.