Scenario: You wish to embed recently uploaded video into your HTML site.
Requirements : Find the Youtube Channel ID
Open the channel and have a look at the url to identify the channel id as shown in below image.
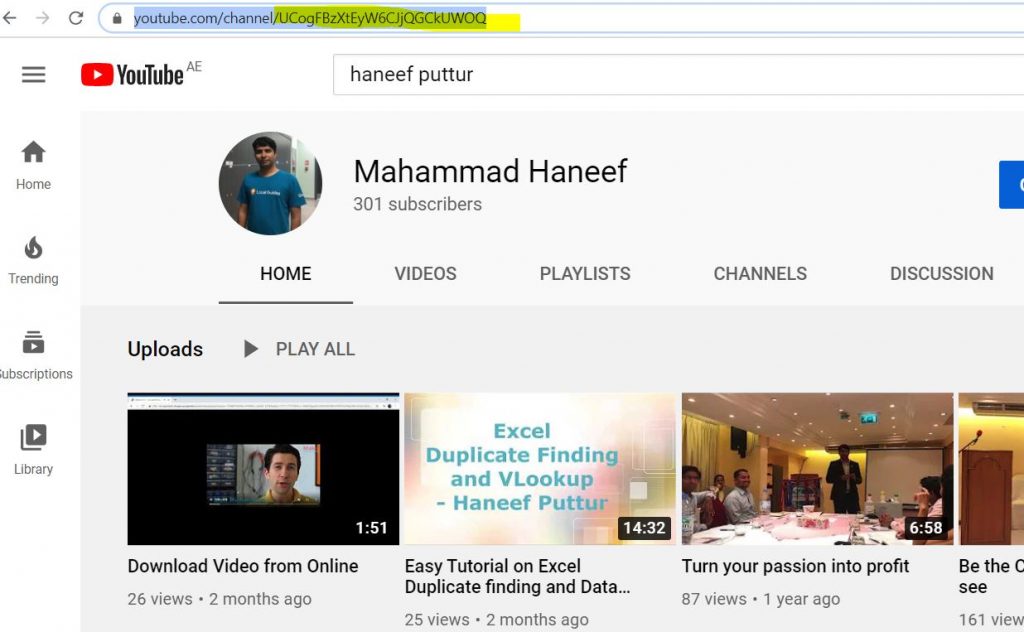
Step 1:
Create as much Div tag you want and name them as 1,2,3 for easy reference.
<div class=”ytube1″></div>
<div class=”ytube2″></div>
<div class=”ytube3″></div>
Step2
Run Ajax query to bring the url of recent videos using below query
$.getJSON('https://api.rss2json.com/v1/api.json?rss_url=https://www.youtube.com/feeds/videos.xml?channel_id=UCogFBzXtEyW6CJjQGCkUWOQ', function(jd) {
$('<iframe width="400" height="315" frameborder="0" allowfullscreen></iframe>')
.attr("src", jd.items[0].link.replace("watch?v=", "embed/"))
.appendTo(".ytube1");
$('<iframe width="400" height="315" frameborder="0" allowfullscreen></iframe>')
.attr("src", jd.items[1].link.replace("watch?v=", "embed/"))
.appendTo(".ytube2");
$('<iframe width="400" height="315" frameborder="0" allowfullscreen></iframe>')
.attr("src", jd.items[2].link.replace("watch?v=", "embed/"))
.appendTo(".ytube3");
});
});
Don’t forget to change to your channel ID.
You can also embed this in any of your web pages. Below is a full sample which i use to embed in one of the site
<html>
<head>
<title>Recent Video from Haneef Youtube</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script>
$(document).ready(function() {
$.getJSON('https://api.rss2json.com/v1/api.json?rss_url=https://www.youtube.com/feeds/videos.xml?channel_iUCogFBzXtEyW6CJjQGCkUWOQ', function(jd) {
$('<iframe width="400" height="315" frameborder="0" allowfullscreen></iframe>')
.attr("src", jd.items[0].link.replace("watch?v=", "embed/"))
.appendTo(".ptryoutube1");
$('<iframe width="400" height="315" frameborder="0" allowfullscreen></iframe>')
.attr("src", jd.items[1].link.replace("watch?v=", "embed/"))
.appendTo(".ptryoutube2");
$('<iframe width="400" height="315" frameborder="0" allowfullscreen></iframe>')
.attr("src", jd.items[2].link.replace("watch?v=", "embed/"))
.appendTo(".ptryoutube3");
});
});
</script>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
* {
box-sizing: border-box;
}
/* Create three equal columns that floats next to each other */
.column {
float: left;
width: 33.33%;
padding: 10px;
}
/* Clear floats after the columns */
.row:after {
content: "";
display: table;
clear: both;
}
/* Responsive layout - makes the three columns stack on top of each other instead of next to each other */
@media screen and (max-width: 600px) {
.column {
width: 100%;
}
}
</style>
</head>
<body>
<div class="row" style="background-color:#eee;">
<div class="column">
<div class="ptryoutube1">
</div>
</div>
<div class="column">
<div class="ptryoutube2">
</div>
</div>
<div class="column">
<div class="ptryoutube3">
</div>
</div>
</div>
</body>
</html>
If you want to pull more video link just refer to index.